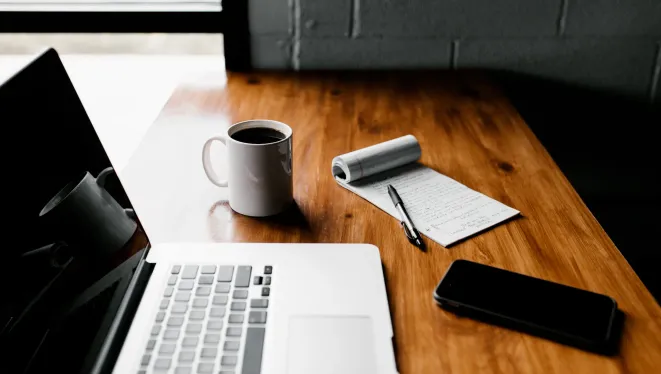
TensorFlow.js Examples
As the world of technology continues to evolve, the integration of machine learning and web development has become increasingly seamless. Delving into the realm of machine learning might seem daunting at first glance. However, with the emergence of tools like TensorFlow.js, the journey becomes not only accessible but also exciting.
TensorFlow.js is a JavaScript library that enables developers to build and train machine learning models directly in the browser or on Node.js. It brings the power of TensorFlow, Google’s open-source machine learning platform, to JavaScript developers, opening up a realm of possibilities for creating intelligent web applications.
Here’s why TensorFlow.js is a game-changer:
Accessibility
TensorFlow.js eliminates the barrier to entry that traditionally existed for machine learning development. Since it leverages JavaScript, a language already familiar to many developers, getting started requires minimal additional learning. You can quickly dive into building machine learning models without having to master new programming languages or frameworks.
Browser Compatibility
One of the most compelling features of TensorFlow.js is its ability to run entirely in the browser. This means that models can be trained and executed directly within web applications, without the need for server-side processing. This capability not only reduces latency but also enhances user privacy by keeping data on the client-side.
Flexibility
TensorFlow.js offers flexibility in model deployment. Whether you’re building a website, a mobile application, or even an IoT device, TensorFlow.js can be seamlessly integrated into your project. Its versatility makes it an ideal choice for exploring machine learning across various platforms and devices.
Educational Value
TensorFlow.js serves as an invaluable educational tool. It provides hands-on experience with fundamental machine learning concepts such as neural networks, data preprocessing, and model training. Through experimentation and exploration, you gain a deeper understanding of machine learning principles while honing your programming skills.
Getting Started
If you are eager to embark on their TensorFlow.js journey, the following steps provide a solid foundation:
Learn the Basics Familiarize yourself with JavaScript fundamentals if you haven’t already. Understanding concepts such as variables, loops, and functions will be essential for working with TensorFlow.js.
Explore Tutorials Numerous tutorials and resources are available online to help you get started with TensorFlow.js. Websites like TensorFlow.js official documentation, TensorFlow.js GitHub repository, and online coding platforms offer tutorials, sample projects, and documentation to guide you through the learning process.
Experiment Don’t be afraid to experiment with different models and datasets. Start with simple projects, such as image classification or regression tasks, and gradually challenge yourself with more complex problems.
Join Communities Engage with the vibrant TensorFlow.js community through forums, social media platforms, and developer meetups. Networking with fellow enthusiasts and experts can provide valuable insights and support throughout your learning journey.
Let’s build a simple neural network
Let’s create a simple example using TensorFlow.js to build a basic neural network that learns to predict the output of a logical OR gate.
Here’s the code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>TensorFlow.js Example: Logical OR Gate</title>
<!-- Import TensorFlow.js library -->
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs@3.11.0/dist/tf.min.js"></script>
</head>
<body>
<h1>Logical OR Gate Prediction using TensorFlow.js</h1>
<div>
<label for="input1">Input 1:</label>
<input type="number" id="input1" value="0">
<label for="input2">Input 2:</label>
<input type="number" id="input2" value="0">
<button onclick="predict()">Predict</button>
</div>
<div id="output"></div>
<script>
// Define the model
const model = tf.sequential();
model.add(tf.layers.dense({units: 1, inputShape: [2]}));
model.compile({optimizer: 'sgd', loss: 'meanSquaredError'});
// Train the model
const xs = tf.tensor2d([[0, 0], [0, 1], [1, 0], [1, 1]]);
const ys = tf.tensor2d([[0], [1], [1], [1]]);
model.fit(xs, ys, {epochs: 200}).then(() => {
console.log('Model trained successfully.');
});
// Function to predict based on user input
function predict() {
const input1 = parseFloat(document.getElementById('input1').value);
const input2 = parseFloat(document.getElementById('input2').value);
const inputTensor = tf.tensor2d([[input1, input2]]);
const outputTensor = model.predict(inputTensor);
const output = outputTensor.dataSync()[0];
document.getElementById('output').innerText = `Prediction: ${output}`;
}
</script>
</body>
</html>
In this example, we’re creating a simple HTML page that allows users to input two binary values (0 or 1) and predicts the output of a logical OR gate based on these inputs.
Here’s a breakdown of the code
- We import the TensorFlow.js library from a CDN to use its functionalities.
- We define a simple neural network model using
tf.sequential()
. The model consists of a single dense layer with one unit, which takes an input shape of [2] because we have two input features. - We compile the model with stochastic gradient descent (‘sgd’) optimizer and mean squared error loss.
- We define training data (xs) and labels (ys) for the logical OR gate. The model is trained using these data pairs.
- When the user clicks the “Predict” button, the
predict()
function retrieves the input values, converts them into a tensor, predicts the output using the trained model, and displays the prediction in the HTML page.
This example provides a basic demonstration of how TensorFlow.js can be used to create and train a simple neural network for performing logical operations.
Conclusion
TensorFlow.js represents a convergence of web development and machine learning, opening up new avenues for innovation and exploration. It serves as a gateway to the fascinating world of artificial intelligence and data science.
By embracing TensorFlow.js, you can develop practical skills, gain valuable insights, and embark on a journey of lifelong learning in the dynamic field of machine learning. As you embark on your TensorFlow.js adventure, remember that every line of code is a step toward unlocking the endless possibilities of intelligent web development.